You’re building a web application. Maybe it’s a live chat platform, a trading dashboard, or a notification system. And right now, you’ve hit that familiar wall—you need it to update in real-time, but all you’re finding are half-explained tutorials, outdated documentation, and endless threads comparing WebSockets, polling, and server-sent events.
Sound familiar?
Real-time functionality is one of those features that sounds simple but gets complicated fast. And let’s be honest—most developers don’t want to manually manage low-level socket connections or spend days figuring out scalability issues. That’s where SignalR comes in. It abstracts away all the gnarly parts of real-time communication and lets you focus on what matters: building a responsive, interactive app that users love.
Whether you’re a solo dev or working with an ASP.NET development company, understanding SignalR can be the difference between an app that feels sluggish and one that feels alive.
In this blog, you’ll learn:
- What SignalR is (and why it’s more than just a WebSocket wrapper)
- How to implement SignalR in ASP.NET Core step-by-step
- How to scale it properly in production
- Real-world use cases where SignalR shines—with actual stats
Let’s break it down.
What is SignalR and Why Should You Use It?
A real-time communication tool for ASP.NET Core is SignalR. It lets your server immediately push material to linked clients; the client need not check the server for changes.
In short, it makes things feel live—think notifications, real-time dashboards, live chats, multiplayer games, or collaborative editing.
But what makes SignalR stand out?
SignalR abstracts the tough parts
Under the hood, SignalR negotiates the best transport method available. If WebSockets are supported (which they usually are), it uses that. If not, it gracefully falls back to Server-Sent Events or Long Polling—so you don’t have to write different implementations for different clients or browsers.
Here’s what SignalR takes care of for you:
- Automatic connection management
- Scalable message broadcasting (with groups)
- Fallback transport mechanisms
- Seamless integration with ASP.NET Core middleware
Basically, it removes a ton of complexity—and that’s why devs love it.
Real-time Communication, Without Reinventing the Wheel
Let’s say you’re building a stock market dashboard. You want prices to update in real-time. You could build your own WebSocket server, handle message broadcasting, and ensure fault tolerance, or you could plug in SignalR, wire up a Hub, and be done in a fraction of the time.
Even better? SignalR is baked right into ASP.NET Core. No weird hacks. No duct tape. Just native integration and clean code.
Stat #1: Real Developer Adoption
According to the Stack Overflow Developer Survey, 47% of .NET developers say SignalR is their go-to solution for building real-time features in web apps. That’s not a fringe tool—it’s industry standard.
And it’s used by big players too: Microsoft Teams, Azure DevOps, and countless SaaS apps rely on SignalR.
So if you’ve been wondering whether SignalR is just a cool experiment or the real deal, there’s your answer.
When You Should Use SignalR
SignalR isn’t for everything. If your app doesn’t need to update instantly, it’s overkill. But here’s when it shines:
- Live chat or messaging apps
- Real-time dashboards (IoT, finance, logistics)
- Collaborative tools (whiteboards, doc editing)
- Live customer support or gaming apps
- Instant notifications (think Facebook or Slack pings)
Basically, if your users expect instant feedback or updates, SignalR is your friend.
Pro Tip: Many teams rely on a trusted .NET development company when building these real-time systems, especially when performance and scale become a concern.
Setting Up SignalR in ASP.NET Core (Step-by-Step)
Though it may seem difficult to include real-time capabilities into your online application, SignalR makes the process really simple—particularly inside the ASP.NET Core environment. This step-by-step analysis will enable you to set up SignalR effectively so you can begin creating live, interactive apps without delving into low-level protocols.
1. Add SignalR to Your ASP.NET Core Project
First, you must include SignalR in your program. ASP.NET Core fully supports SignalR; depending on your .NET version, you may include it as a NuGet package or reference it directly.
Once included, SignalR becomes simply another service integrated into your application's dependency injection mechanism.
Key benefits:
- Native support within ASP.NET Core
- Fast installation and configuration
- Well-maintained and updated by Microsoft
2. Create a SignalR Hub
A Hub is a class that handles the server-to-client communication. It serves as a real-time conduit over which your backend may transmit and receive messages to and from clients.
Here is where you specify the real-time communication logic for your app, including message broadcasting, notification sending, and group user updating.
With a Hub, you can:
- Call methods on connected clients
- Receive messages from users and respond instantly.
- Organise clients into groups for targeted broadcasting.
3. Configure SignalR in the Middleware Pipeline
Once the Hub is in place, it needs to be registered within the application’s routing system. This step ensures that your app knows how to handle real-time requests and directs them appropriately to your Hub.
It’s important to:
- Register the service in the Startup or Program class
- Define the route where clients will connect.
- Ensure SignalR runs alongside your existing MVC or API pipeline.
4. Connect the Client Side
With the server set up, the next step is enabling your clients to establish a connection.
SignalR supports:
- JavaScript/TypeScript clients for web applications
- .NET clients for desktop and backend systems
- Mobile clients using Xamarin or MAUI
This flexibility makes SignalR ideal for building cross-platform, real-time experiences.
Stat #2: Faster Deployment Speeds
According to the 2023 DevSurvey, developers using SignalR reported a 65% faster time-to-deployment compared to those implementing WebSocket solutions manually. SignalR's abstraction of transport negotiation, fallback mechanisms, and reconnection handling significantly reduces development time.
5. Secure Your SignalR Implementation
Security should never be an afterthought—especially in real-time apps that deal with sensitive data or user interactions. Ensure your setup includes:
- Authentication and authorisation for Hub access
- Transport encryption via HTTPS
- Connection throttling for heavy loads
- CSRF protection where necessary
Consulting a competent ASP.NET development company might be beneficial if you intend to expand your software or include SignalR into a big system. Working with a company that provides certain ASP.NET development services ensures that your application is created with scalability, security, and performance in mind.
Best Practices for Building Scalable Real-Time Apps with SignalR
Getting your SignalR setup working in development is one thing, but ensuring it scales smoothly under real-world load is another. Real-time applications, by nature, deal with high-frequency, bidirectional communication. That means careful planning is essential if you want your app to perform reliably at scale.
Below are key best practices to follow when building scalable real-time applications using SignalR in ASP.NET Core.
1. Use Backplane for Load-Balanced Environments
If your application runs on multiple servers (which it should if you're preparing for scale), you’ll need to ensure messages are shared across all instances. That’s where a SignalR backplane comes in. It acts as a central message bus, ensuring connected clients receive updates no matter which server they’re connected to.
Recommended backplanes:
- Azure SignalR Service (fully managed)
- Redis (widely used in on-prem or cloud-native apps)
- Azure Service Bus (for complex, event-driven architectures)
Why this matters: Without a backplane, users connected to different server instances won’t receive consistent messages, breaking the real-time experience.
2. Optimise Message Payloads
SignalR makes it easy to send data, but that doesn’t mean you should send everything. Keep your payloads light to reduce latency and bandwidth usage.
Tips:
- Avoid sending unnecessary metadata
- Compress large payloads
- Use strongly typed objects for clarity and consistency.
- Limit update frequency—don’t overwhelm clients with updates every few milliseconds.
Use case: In live dashboards, only push changes that affect what's displayed—there’s no need to resend the full dataset every time.
3. Monitor Connections and Performance Metrics
Real-time apps need continuous observability. Monitoring client connections, message delivery rates, and errors helps you detect issues early—before users complain.
What to monitor:
- Active connections
- Message delivery times
- Disconnections and reconnects
- Server resource usage (CPU, memory, network I/O)
Many teams integrate SignalR with Application Insights, Prometheus, or custom dashboards to keep an eye on system health.
4. Scale with Azure SignalR Service (Optional but Powerful)
If you’re deploying in the Azure ecosystem, consider using Azure SignalR Service. It’s a fully managed, production-ready service that handles connection scaling, backplane management, and load balancing automatically.
Benefits:
- Scales to thousands (or millions) of clients
- Handles transport fallback and connection state
- Integrates with other Azure services (like Functions, Event Grid, and Cosmos DB)
Many growing tech teams partner with a trusted .NET development company when scaling complex real-time systems. A partner can help ensure your infrastructure is both robust and cost-effective.
Stat #3: Consistent latency under 100ms was shown by a Microsoft internal benchmark to be handled by Azure SignalR Service up to 1 million concurrent connections, making it perfect for high-scale applications such as live financial dashboards or multiplayer gaming.
Common Challenges When Using SignalR (And How to Solve Them)
Though it's a great tool for real-time communication, SignalR, like any other technology, has its own difficulties, particularly if you're running production settings or handling scale. Should you be developing or managing a real-time ASP.NET Core application, you will most likely encounter one of them eventually.
Here are the most typical obstacles programmers encounter using SignalR and how to address them.
1. Unstable Connections
Real-time means instant, but unstable internet connections or misconfigured timeouts can disrupt that experience. If clients are getting disconnected often, it’s not just frustrating—it’s a usability issue.
How to handle it:
- Enable automatic reconnection on the client side
- Set appropriate keep-alive intervals on the server.
- Monitor disconnections to identify patterns (e.g. mobile users or poor regions)
Bonus tip: If you're using WebSockets, ensure your hosting environment and reverse proxies (like NGINX or IIS) are configured to support long-lived connections.
2. Debugging Message Flow
When something breaks, tracing message flow across clients and the server can be tricky. Messages might not arrive, arrive twice, or get delayed—and you’ll need visibility into the pipeline to diagnose it.
Best practices:
- Log all message sends and receives for critical communication points
- Use tools like SignalR logging with Application Insights.
- Always test in both single-user and multi-user scenarios to catch edge cases.
3. Scaling Without a Strategy
You might start with a simple setup, but once your app has thousands of users, poor planning can lead to lag, dropped messages, or server overloads. Scaling needs to be baked in early, not added later in panic mode.
What to do:
- Use a backplane or Azure SignalR Service for scaling across servers
- Implement connection limits and message throttling.
- Consider using sticky sessions or distributing users into logical groups.
And if scaling feels complex, it’s worth engaging professional ASP.NET development services to ensure you're not reinventing the wheel.
4. Security Missteps
SignalR introduces a persistent connection between the client and server, which can create new vulnerabilities if left unguarded. Authentication and authorization should never be an afterthought.
Secure your app by:
- Requiring authentication for all Hub connections
- Authorising access to specific methods or groups
- Ensuring HTTPS is enforced and TLS is enabled
- Validating inputs—SignalR is still exposed to injection attacks and payload abuse.
Stat #4: Real-Time Isn’t Optional Anymore
According to Stack Overflow’s 2023 Developer Trends Report, over 68% of developers consider real-time functionality a core requirement in modern web applications, especially for features like live chat, notifications, and collaborative editing.
Conclusion
Users increasingly demand instantaneous updates, live alerts, and smooth collaboration in real-time online apps, which are no longer only "nice to have." SignalR in ASP.NET Core provides a simple, strong approach to creating these experiences without getting into the details of low-level WebSocket management.
It's tightly integrated with ASP.NET Core, easy to set up, and scalable, utilizing technologies like Azure SignalR Service or Redis backplanes. Performance and dependability, therefore, relies on intelligent design decisions, including payload optimization, connection monitoring, and communication security.
Although SignalR is strong, it's not always plug-and-play; hence, from day one, it's crucial to consider scalability and cross-platform compatibility. Working with a knowledgeable ASP.NET development business or a team providing ASP.NET development services will help you create a safe, scalable solution if you don't know where to begin.
SignalR is more than capable of managing the real-time needs of your application, whether you are building a trading dashboard, a collaborative whiteboard, or live chat.
Ready to make your concept a reality? Work with a reliable .NET development company to eliminate technological obstacles as you expand.
FREQUENTLY ASKED QUESTIONS (FAQs)
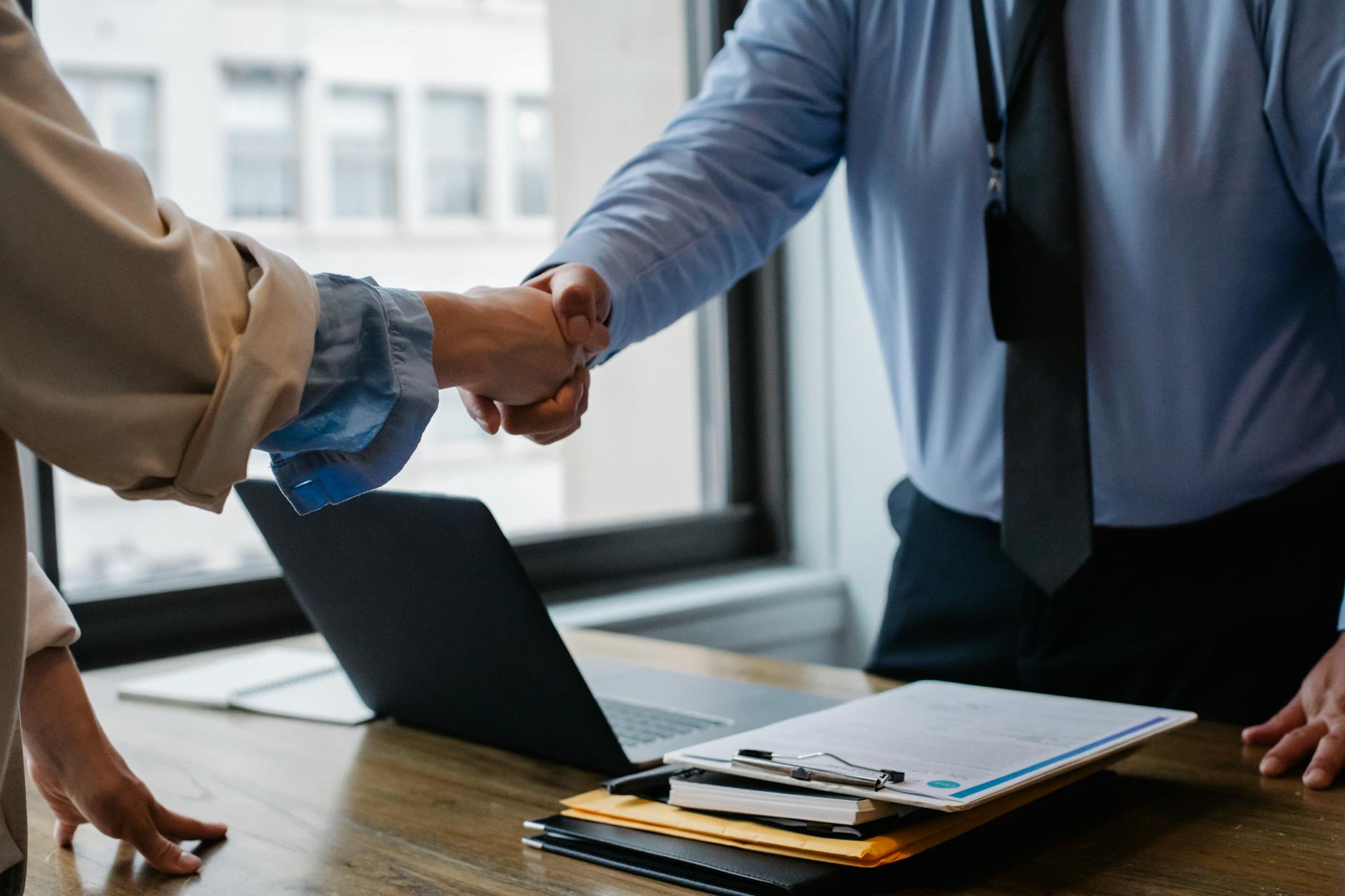