- What Does Offline-Capable Really Mean for iOS Apps?
- When and Why to Build Offline-First Apps
- Key Architecture Patterns for Offline-Capable iOS Apps
- Storing Data Locally: Core Data, SQLite, or Realm?
- Handling Sync: How to Reconnect & Push Data Safely
- Detecting Network Status – Reachability, NWPathMonitor, and More
- Error Handling and UX Tips for Offline States
- Tools, Libraries, and Frameworks for Offline iOS Development
You tap to open an app. Nothing loads. You’re offline, and the app is useless.
It’s a small failure that breaks user trust fast. And in today’s mobile world, it’s one you can’t afford.
According to Google’s Mobile UX report, 53% of users abandon apps that fail or crash when offline, even once.
Yet, many iOS apps are built assuming constant connectivity. But users don’t always have it —they’re on planes, trains, elevators, or in rural areas. If your app can’t handle that, you’re not building for the real world.
The good news? Offline support on iOS isn’t just possible — it’s totally doable with the right architecture and tools.
In this guide, you’ll learn exactly how to build an offline-capable iOS app: how to store data locally, detect connection changes, handle sync, and deliver a smooth user experience that works — even when the network doesn’t.
Let’s build an app that won’t break when your users need it most.
What Does Offline-Capable Really Mean for iOS Apps?
An “offline-capable” app isn’t just one that doesn’t crash without Wi-Fi — it’s one that actually functions when the network drops.
In practical terms, an offline-capable iOS app should let users perform key actions even when disconnected, like viewing recent content, drafting new entries, saving form data, or browsing cached pages. When connectivity returns, the app syncs in the background without errors or data loss.
But offline support isn’t binary — it’s a spectrum. Some apps need read-only offline access (like news readers), while others require read-write offline capabilities (like note-taking, CRM, or field service apps).
Here’s what offline functionality often includes:
- Local data storage (via Core Data, SQLite, or Realm).
- Intelligent caching of recent content or screens.
- Background sync logic to handle reconnection cleanly.
- Network awareness using Reachability or NWPathMonitor.
- User feedback for offline/online states (e.g., toast messages, indicators).
- Conflict resolution for data changes made offline.
Offline capability is more than a feature — it’s a reliability signal. Users may not notice perfect offline support right away. But they’ll notice instantly if it’s missing.
When and Why to Build Offline-First Apps
Not every app needs offline support, but a surprising number do.
If your app deals with user-generated content, data entry, or fieldwork, there’s a strong case for building it to work offline first, not as an afterthought.
An offline-first iOS app is one that’s designed with intermittent connectivity in mind. It assumes the network may fail and ensures the core experience still works when it does.
So, when should you go offline-first?
- Your users are mobile or remote. Think delivery drivers, field techs, travellers, or workers in rural areas.
- Your app handles critical tasks. Medical, logistics, or productivity apps can’t afford to “break” without internet.
- You need fast, consistent performance. Fetching everything from the network adds latency — local-first design makes the app snappier.
- Your users expect reliability. If people rely on your app for work, communication, or time-sensitive tasks, offline support builds trust.
Why it matters:
Apps with offline support have 2x higher retention among users who travel or work on the move. (Source: Localytics Mobile Usage Report, 2023)
Offline-first development isn’t just a developer challenge — it’s a competitive advantage.
Key Architecture Patterns for Offline-Capable iOS Apps
Building an offline-capable app starts with the right architecture. You’re not just storing data —you’re managing sync, local writes, and recovery from disconnection. The goal? A reliable, fault-tolerant app that doesn't rely on being constantly online.
One of the most common patterns is the offline-first + sync-on-connect model. Here, the app writes to local storage first (using Core Data, SQLite, or Realm), queues changes in a local “outbox,” and syncs with the server once a stable connection is detected. It’s simple, effective, and scalable.
For more complex apps, event sourcing or command queue architectures work well. These patterns track every data change or user action, apply them locally, and then replay them on the backend when reconnected — useful for apps with many users editing shared data.
You’ll also want to separate your data layer from the UI — often with MVVM or Clean Architecture — so offline logic doesn’t clutter views or controllers. And finally, don’t forget to build your sync layer to be retryable and idempotent, so failed requests don’t corrupt data or duplicate entries.
The right architecture can make offline support feel seamless, and partnering with an experienced mobile app development company will lead you onto this path.
Storing Data Locally: Core Data, SQLite, or Realm?
When your app goes offline, local data storage becomes your foundation. The question is: which tool should you build on?
iOS offers several strong options, each with its own pros and trade-offs. Here’s a breakdown of the most commonly used:
1. Core Data (Apple’s Native ORM)
- Best for apps with structured, relational data.
- Deep integration with Swift and SwiftUI.
- Supports background syncing and lightweight fault-tolerance.
- A bit verbose, with a steeper learning curve.
Ideal for: Complex iOS apps that need tight system integration or advanced querying.
2. SQLite
- A lightweight SQL-based storage engine.
- Offers full control over your schema and queries.
- Very stable and predictable.
- Requires manual boilerplate and sync logic.
Ideal for: Developers who prefer SQL and need low-level control or cross-platform sharing.
3. Realm (Now owned by MongoDB)
- Easy setup with reactive data-binding.
- Good performance for offline use.
- Automatic syncing with MongoDB Atlas (optional).
- Less native than Core Data in some areas.
Ideal for: Apps that need fast, flexible local storage with optional cloud sync built in.
Handling Sync: How to Reconnect & Push Data Safely
Building offline features is only half the equation — the real challenge begins when your app reconnects. You need a smart, resilient sync strategy that pushes data to the server without losing user changes or overwriting critical information.
The safest approach is a sync-on-connect flow. When the app detects a stable connection, it begins syncing a queue of locally stored actions or updated records. You’ll want this logic to run in the background using background tasks or silent push notifications (especially with BackgroundTasks in iOS).
But the key here is reconciliation. You need to track what was changed offline and compare it with what’s now on the server. Timestamps, change tokens, or versioning flags are essential for avoiding data conflicts.
For apps where multiple users can update the same record, conflict resolution becomes critical. You can choose from:
- Last-write-wins (simple, but risky).
- Merge strategies (smart merging of changes).
- Manual conflict resolution (user gets a “Review conflict” prompt).
Also, ensure your sync logic is idempotent — meaning it can retry safely without creating duplicate records.
If your app syncs too aggressively or without safeguards, you risk corrupting data or creating hard-to-debug bugs. Sync should be tested under flaky network conditions, not just in perfect scenarios.
Detecting Network Status – Reachability, NWPathMonitor, and More
When building offline-capable apps, detecting network status changes is essential. You need to know when the user’s device is online or offline so that you can trigger the right sync actions, alert the user, or handle any errors.
There are a few key tools in iOS for network status detection:
1. Reachability (Legacy)
Reachability has been a long-standing tool for detecting whether the device is connected to the internet. It uses Apple's SystemConfiguration framework to monitor network changes. While it works well for basic scenarios, it is deprecated in newer iOS versions, and it’s best to consider other options moving forward.
2. NWPathMonitor (Preferred in iOS 12 and Later)
NWPathMonitor is Apple’s modern, preferred tool for network status monitoring, introduced in iOS 12. It offers a more powerful and flexible API to track real-time network changes, such as switching from Wi-Fi to cellular.
With NWPathMonitor, you can:
- Monitor changes in network connectivity (Wi-Fi, cellular, or none).
- Detect whether a device is connected to the internet (not just to a local network).
- Handle connectivity updates with greater accuracy and control.
3. Network Extension API (Advanced)
For more granular control over network interfaces and detailed connection management (e.g., for VPN apps or network-centric tools), Apple provides the Network Extension API. This is advanced but necessary for specific app use cases.
By using NWPathMonitor for detecting network status in modern iOS apps, you can ensure your offline handling logic is tightly integrated with network changes — ensuring a smooth, fault-tolerant experience for users.
Error Handling and UX Tips for Offline States
The last thing you want is for users to feel irritated or perplexed when they are offline. Delivering a smooth, user-friendly experience depends on handling offline conditions gracefully; this also guarantees the software seems dependable even if connectivity fails.
Here’s how to handle offline states and errors effectively:
1. Show Clear Offline Indicators
Always inform users when they’re offline. Demonstrate a straightforward, discrete message or icon, such as a "No connection" icon, that lets people know they're operating offline. Users won't feel lost if this is a status bar or a little banner at the top of the screen.
2. Allow Data Editing in Offline Mode
Users should still be able to interact with the app, even when offline. Let them edit data, fill out forms, or draft new content while offline. Store these changes locally and sync them when the device is back online. For example, note-taking, e-commerce carts, and email apps benefit from this.
3. Provide Retry Mechanisms
If syncing fails due to network issues, offer a retry option. Automatic sync when connectivity picks up and retry options help to guarantee data isn't lost. Make sure the retry function is idempotent as well; it should safely manage several retries without generating duplicate data.
4. Gracefully Handle Sync Failures
Should there be sync problems, inform users. Display a clear error notice with practical steps, like “Retrying in 30 seconds” or “Unable to sync data. Please check your connection.” This feedback reduces user anxiety and helps them understand what’s going on.
5. Limit Data Usage When Offline
When possible, reduce the amount of data the app stores or syncs when offline. Prioritize essential content to prevent performance issues or excessive data usage during sync. For example, load essential text but delay heavy images or videos until the app is back online.
One where people don't feel trapped or isolated is a smooth offline experience. Reducing friction and fostering confidence that the program will run well regardless of connection status defines it.
Tools, Libraries, and Frameworks for Offline iOS Development
The vast ecosystem of tools, libraries, and frameworks available makes building offline-capable iOS apps easier than ever. These tools provide developers a head start in creating strong offline capability by simplifying chores such as data storage, synchronization, and network change detection.
Here are some of the most useful iOS offline development tools:
1. Core Data
Core Data, Apple's native object graph and persistence system, is ideal for organizing and storing offline data. Perfect for applications with structured data that must be kept offline, it enables data syncing, local caching, and sophisticated searches. For background syncs, Core Data can operate flawlessly with NSPersistentContainer.
2. Realm
Realm is an open-source mobile database designed for offline-first iOS apps. It’s easier to use than Core Data, and it offers better performance for certain use cases. Realm also provides automatic sync capabilities with MongoDB Atlas but can function entirely offline if needed. Perfect for apps with simpler data structures or rapid iteration cycles.
3. SQLite
For mobile devices, SQLite is a strong, lightweight relational database. It's ideal when you need direct control over your data storage and schema. Although it requires more boilerplate than Realm or Core Data, it’s fast, stable, and offers full SQL querying capabilities.
4. Firebase Realtime Database & Firestore
Although mostly for cloud-based databases, Firebase also enables offline operation. For instance, Firestore keeps data locally on the device and syncs it with the cloud if a network connection becomes available. For offline-first applications, Firebase provides great real-time synchronization capabilities.
5. Reachability & NWPathMonitor
For detecting network changes, Reachability and NWPathMonitor (for iOS 12+) are indispensable. These libraries let you know when the device goes offline and back online, hence enabling your app to respond appropriately, whether by halting sync, caching new data, or displaying an offline mode indicator.
6. Alamofire
A strong networking tool, Alamofire streamlines network queries including those for offline use. A fantastic choice for applications requiring offline syncing and retry techniques, it can handle automatic retries and control network status changes in concert with Reachability or NWPathMonitor.
7. Couchbase Mobile
Couchbase provides Couchbase Lite, a mobile-first database with sync features. For offline-first apps, it is a fantastic option since it enables smooth data syncing when network conditions are restored. It also offers real-time data synchronization with Couchbase Server and full-text search.
These tools and libraries enable you to implement offline functionality effectively, ensuring your iOS apps are reliable and performant, no matter where your users are.
Conclusion: Building Reliable Offline-Capable iOS Apps
Building offline-capable iOS apps is not only a nice-to-have in a mobile-first society; it's a need. Users expect consistent performance and dependability, even without a network, whether your app is for travel, e-commerce, or healthcare. No matter where your users are, you can guarantee your app runs well by using strong tools like Core Data, Realm, and Reachability and applying the appropriate offline-first tactics.
The idea is to emphasize local data storage, smart sync techniques, and offering unambiguous feedback when offline. The correct architecture and technologies will enable you to design a smooth, user-friendly experience that functions perfectly in both online and offline settings.
Partnering with an experienced iOS development company will allow you to bring your vision to life—from concept to deployment if you are ready to take the next step in creating a strong offline-capable iOS app.
FREQUENTLY ASKED QUESTIONS (FAQs)
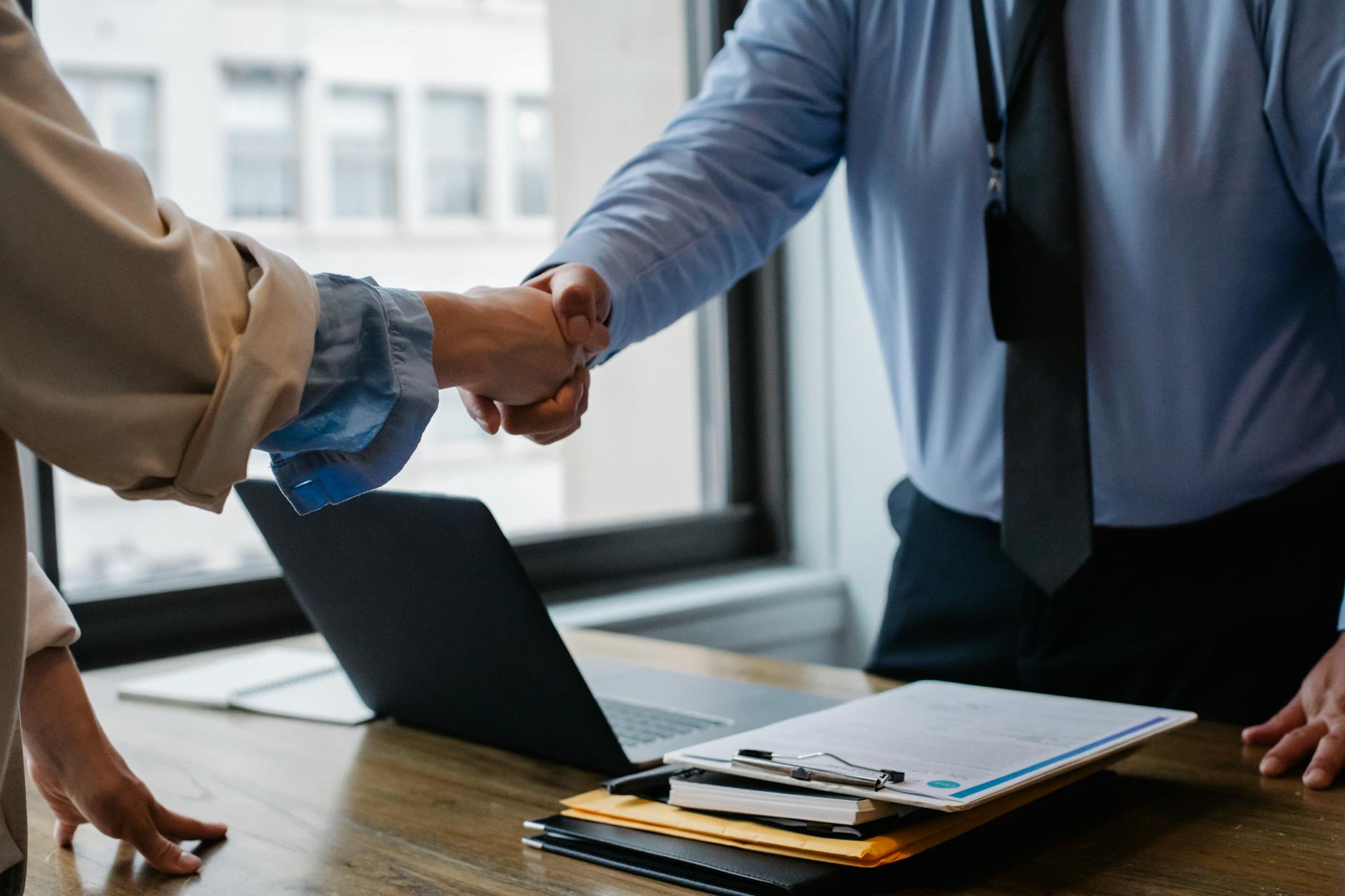